Intoduction to ROS
Goal: Understanding the general concept of ROS framework
What is ROS?
If you ever had any chance to develop code for robot or taking part in a robotic team, you have definitely heard of ROS. The Robot Operating System (ROS) is the most used and flexible framework for writing robot software. It is a collection of tools, libraries, and best practices to simplify the task of creating complex and robust robot application. In a nutshell, ROS uses distributed publish and subscribe architecture where different software components communicate with each other via messages. Distributed architecture means that your program which is running on a machine (e.g. your PC or a Tablet) can communicate with and commands a second program running on another machine (e.g. on the robot). Taking a deep dive into ROS software architecture is beyond the objective of this blog. Nevertheless I will explain its main concepts using simpler words and examples in the following sections.
Topics and Messages
You may still recall online chatrooms from some late 90s chat programs. People, who were interested in specific topic used to show up in a relevant chat room and talk with each other by exchanging messages. ROS topics and messages work in a similar manner. A software program can show interest in a specific topic by subscribing to its channel or advertising a channel on a new topic. Via these channels, programs then can communicate by exchanging messages.
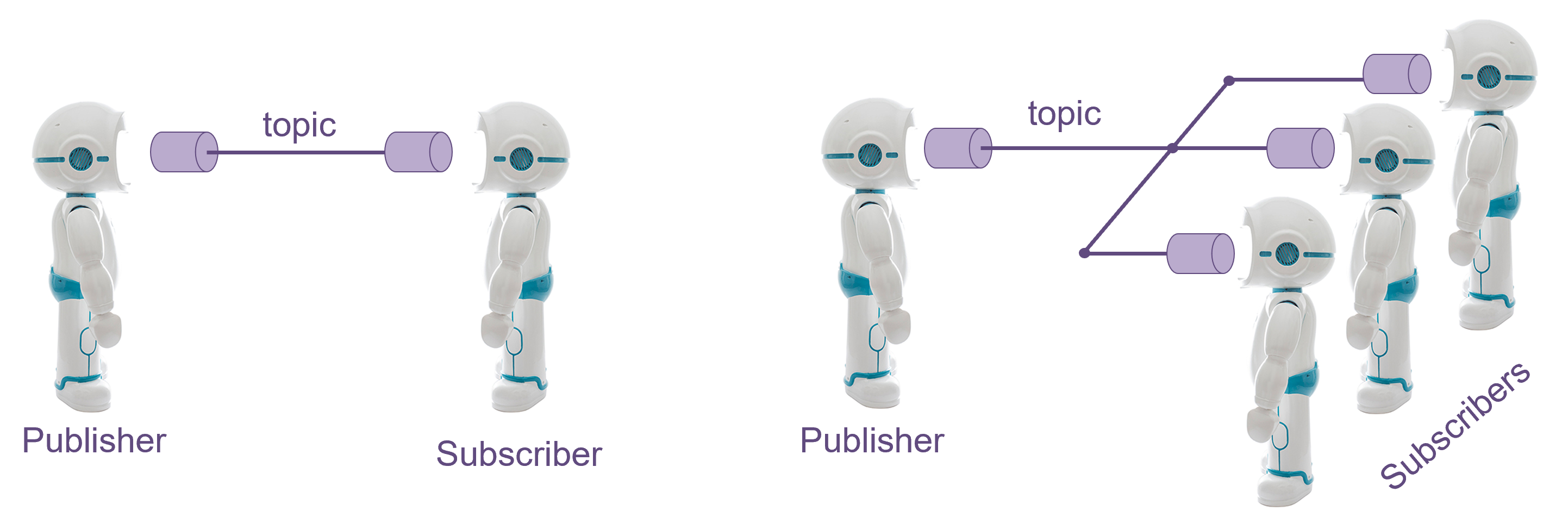
To understand each other, people must speak the same language in a common chatroom. Likewise, ROS programs must also know the type of the message used in specific topic. For example, QTrobot advertises a topic called /qt_robot/speech/say
which uses message of type std_msgs/String
. It is very common and standard message type in ROS which represents simple String (text) message. Messages can have more complex type which composed of different simpler messages. For example, /qt_nuitrack_app/faces
topic uses a custom message of type qt_nuitrack_app/FaceInfo
. This message represents a collection of human facial features such as emotions (e.g. happy, angry, surprised), the person estimated age, eyes gazing angles, etc. You may want to take a look at the List of available topics which are advertised by QTrobot.
Publishers and Subscribers
Consider again our chatroom example. The person who is writing a message is the publisher and those who are reading the message are subscribers. One or multiple publishers can send (publish) messages on the same topic. Similarly multiple subscribers can receive messages from the same topic.
Service calls
The publish-subscribe model that we have explained is a very flexible communication paradigm. However, it is a on-way communication: one talks and the others listen. Indeed, it is possible to establish two way communication by letting each entity becomes publisher and subscriber at the same time, but as you can imagine this may get chaotic (especially when everyone wants to talk simultaneously) and become tedious to be synchronized with each other. We need something like request-response model: one requests something from an entity and wait until the other replies. Let's consider pizza ordering scenario: there is a restaurant which provides pizza delivery service. the name of this restaurant is Pizzeria. We make a call and request for a pizza. If the restaurant offers only one type of pizza, then we do not need to provide them any more information, but in most cases we need to let them know at least which type of pizza we would like. We may even want to further customize our order by choosing among different topping options (parameters) they offer. Then all we need is to wait until the restaurant send us our pizza.
ROS services works in similar fashion. A software program provides and advertise specific type of service along with required parameters. Others programs can call that service and get the response. For example, QTrobot provides a service called /qt_robot/speech/config
to configure the robot speaking language. The type of this service is qt_robot_interface/speech_config
and has some parameters such as language
and speed
. Another programs can call this service to change the robot's speaking language and talking speed at the run time.
QTrobot ROS Topics and Services
To get the list off all Topics or Services you can run one of this commands:
Topics:
rostopic list
Services:
rosservice list
QTrobot speech interface
Let's start with QTrobot Speech interface. If you do rostopic list
you will see that one of the topics is /qt_robot/speech/say
. If we publish to that topic QTrobot will say the text message that we wrote. Open the terminal and try this:
Publisher:
rostopic pub /qt_robot/speech/say std_msgs/String "data: 'Hello I am QT'"
Service call:
rosservice call /qt_robot/speech/say "message: 'Hello I am QT'"
QTrobot talk text interface
The /qt_robot/behavior/talkText
interface is similar to /qt_robot/speech/say
interface with the only different that the talkText interface asks QTrobot to move his lips while reading the text messages. To try it, just add the following lines to our code and look at the QTrobot's face:
Publisher:
rostopic pub /qt_robot/behavior/talkText std_msgs/String "data: 'Hello I am QT'"
Service call:
rosservice call /qt_robot/behavior/talkText "message: 'Hello I am QT'"
QTrobot emotion interface
Now lets show an emotion on QTrobot face. QTrobot comes with plenty of predefined emotion animations. You can find the complete list of the available emotions either using the QTrobot Educator app or by looking into the ~/robot/data/emotions
folder in QTRP.
Publisher:
rostopic pub /qt_robot/emotion/show std_msgs/String "data: 'QT/happy'"
Service call:
rosservice call /qt_robot/emotion/show "name: 'QT/happy'"
As it shown in the above example, you should not give the emotion's file extension (.avi
) to the interface!
QTrobot gesture interface
Now lets play a gesture with QTrobot. QTrobot comes with plenty of predefined gestures. You can find the complete list of the available gestures either using the QTrobot Educator app or by looking into the ~/robot/data/gestures
folder in QTRP.
Publisher:
rostopic pub /qt_robot/gesture/play std_msgs/String "data: 'QT/happy'"
Service call:
rosservice call /qt_robot/gesture/play "name: 'QT/happy'
speed: 0.0"
rosservice call /qt_robot/gesture/play "name: '' speed: 0.0"
As it shown in the above example, you should not give the gestures's file extension (.xml
) to the interface!
QTrobot audio interface
Now lets play an audio file on QTrobot. QTrobot comes with some audio examples. You can find the complete list of the available audios either using the QTrobot Educator app or by looking into the ~/robot/data/audios
folder in QTRP. QTrobot can play both audio wave and mp3 files.
Publisher:
rostopic pub /qt_robot/audio/play std_msgs/String "data: 'QT/Komiku_Glouglou'"
Service call:
rosservice call /qt_robot/audio/play "filename: 'QT/Komiku_Glouglou'
filepath: ''"
As it shown in the above example, you do not need to give the audio's file extension (.wav
or .mp3
) to the interface!